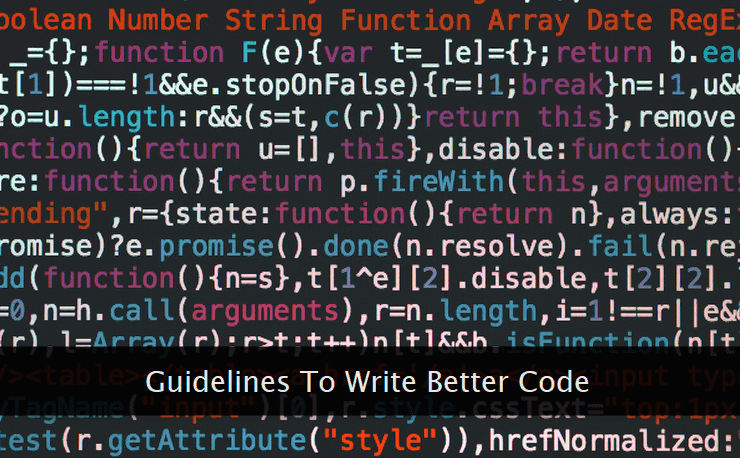
It's up to you how you use it in your coding practice. So, here we go and see how we can write production code that not only helps you build great software but also help your peers better understand it.
Ensure Readability
No matter how efficient and optimized code you've written, unless it is not formatted well, it's just crap for other developers. Alignment, indentation, and spacing are equally important as the code itself.Readability adds value to your code and makes it much more extensible. You can use a prettifier plugin for your favorite source code editor. The better the readability of your code, the better you and your peers can work on it without any hiccups.
Use Annotations (Comments)
Proper code formatting alone doesn't make your code easily understandable unless you compliment it with annotations. Almost every language supports inline comments to add helpful annotations to the code.This is very helpful when you're working with a team and your code is used by other members of the team. Annotations ease the deciphering process and help in quickly absorbing the functioning of your code by other programmers.
Create Documentation
Although annotation is a big help in deciphering the code, still it does not give a larger picture in one go. And that's where technical documentation comes in handy. Whether it's flowcharts, rough sketches or notes, decision tables, or pie charts, keeping a hard or soft copy of these documents helps a great deal in a better understanding of your code.And above all, documentation acts like a raw framework that presents a big picture giving you enough idea about how all pieces are knitted together to get a finished product. You can also use specialized software documentation tools like Doxygen or JSDoc to quickly generate professional-grade technical documentation for your code.
Use Version Control System
Large projects involving a continuous development routine require proper archiving of source code as well as the builds (executable) for later reference. Unless you maintain each and every version of your source code files, you'll struggle to refer things while going back in the development tree.You can use a specialized version control system like Git to archive each version of your source code files. This way you can easily trace the development path for any feature of your software.
Reduce Dependencies
Now, this can be a bit tricky. Depending on the platform and software you're using, reducing external dependency (external libraries) may enhance the performance and efficiency of your application.If you choose the cut down external dependencies drastically, your application's executable can become quite large and may consume a large amount of memory.
On the other hand, if you keep only the necessary core routines in the main executable and connect with external libraries dynamically during run-time, it may consume less memory but performance may degrade significantly. It's up to you to assess and formulate a strategy about which external dependencies will be fine for the application.
Delay Module Loading
Dynamically linking with an external library is one of the common traits of modern applications. This technique doesn't load external modules in memory until they're not needed by the application. It may happen that your application rarely needs an external dependency during the run-time.This saves the system's memory and keeps the application fast and efficient. Carefully plan the load of dynamic libraries and make sure all rarely used libraries are only loaded when they are actually required during run-time.
Ensure Zero Memory Leaks
One of the problems with poorly coded applications is memory leakage that brings the system down while performing critical tasks. Unfortunately, very fewer programmers check for memory leaks.Checking for memory leaks is generally done thoroughly during the testing phase through artificial test cases. Make sure all memory allocations are relinquished in your source code through at least one condition.
Implement Exception Handling
Applications may behave unexpectedly on the client's site or on end-user machines. During the development phase, no matter how good are your test cases, you cannot emulate almost every possible condition while testing the software.To prevent crashing of your software in exceptional conditions, make sure you thoroughly implement exception handling logic in your source code. Good software has 25% to 30% business logic and the rest part comprises exception handling.
Optimize for Less CPU Usage
In the programming world, you can code the same task in 10 different ways. Writing problem-solving code and writing efficient problem-solving code are two different things. Do not settle for the very first code sequence written for solving a given problem.Do some research and testing to find out the most efficient way to get things done. Your goal should be to make an application that is memory efficient and consumes fewer CPU resources. This way your refactored software will not be categorized as memory or resource hogger.
Add User-Friendly Help Messages
While developing software, do take care of coding help messages through popups or tool-tips wherever required. Good software without such help messages is like an egg without an outer shell.User-friendliness is one of the major features of good software. While programming such help messages, keep the message text in memory rather than pulling from the back-end.